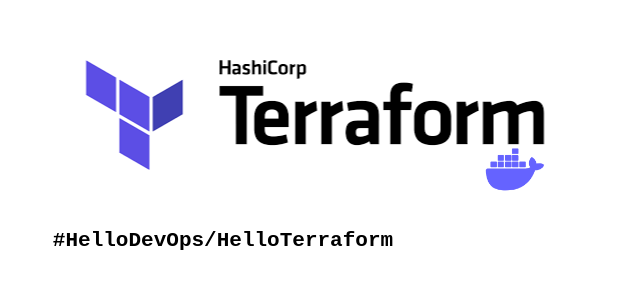
Are you just starting to dive into the world of infrastructure automation and looking for a powerful tool to help you manage your resources? Look no further! In this beginner-friendly blog post, you’ll learn the basics of Terraform and how to use it with Docker to automate your infrastructure setup. With clear explanations and step-by-step tutorials, you’ll be up and running with Terraform in no time.
If you want to dive right into the code, head over to the HelloTerraform repository on GitHub to check out the Terraform configuration that sets up a Docker environment!
1. What’s Terraform?
Terraform is a cool tool that helps you build and manage your infrastructure easily. It’s open source and lets you write down your infrastructure setup like a code. It was made by HashiCorp and has been around since 2014. People really like using Terraform because it’s easy to keep track of changes and share with others. And the best part? You can use it on different clouds, like AWS, Google Cloud, and Microsoft Azure, or even on your own computers. All from one place!
2. Install Terraform
Getting Terraform on your computer is easy! You can use it on Windows, Linux, or macOS. You have a few choices for getting it - you can download it from the HashiCorp website, build it yourself, or use a package manager. If you’re using Windows, just go to the Terraform website, choose the right version for your computer, and download the file. Then, add Terraform to your computer’s PATH
so you can run it from the command line.
For Linux systems, Terraform can be installed using a package manager, such as apt
for Ubuntu systems or yum
for CentOS systems. You can also download the Terraform binary from the HashiCorp website and install it manually.
For those who prefer to use Docker, Terraform is available as a Docker image. To use Terraform with Docker, you can simply run the Terraform image and pass in your Terraform configuration as a volume. This allows you to use Terraform in a containerized environment, making it easy to manage and share Terraform installations.
1
docker run --name terraform -it -v $(pwd):/app hashicorp/terraform:0.X.Y
In these examples, replace “0.X.Y” with the version of Terraform that you want to install. The latest version of Terraform can be found on the HashiCorp website.
3. Terraform
Docker
Terraform and Docker are a match made in heaven! Terraform helps you set up your infrastructure, and Docker helps you run your applications in containers. When you use Terraform with Docker, you can manage your entire infrastructure, including your Docker containers, with ease.
Here’s how it works? Terraform can manage the creation and configuration of your Docker containers, and then launch those containers within your infrastructure. This makes it easy to automate your entire application environment, from the underlying infrastructure to the containers running your apps.
Using Terraform with Docker also allows you to manage multiple environments, like development, testing, and production, in a consistent and repeatable manner.
4. Start with the Terraform configuration file
Ready to start with Terraform? The first step is the Terraform configuration file! This file is like a blueprint for your infrastructure and tells Terraform exactly what you want it to do. The file is written in a language called HashiCorp Configuration Language (HCL
) and has all the details about the resources you need, like servers, databases, and networks.
Think of it this way: the Terraform configuration file is like a map that helps Terraform navigate and set up your infrastructure just the way you want it. And don’t worry if HCL is new to you, it’s super easy to learn and you’ll be a pro in no time!”
So what exactly goes into the Terraform configuration file? There are three main components:
- Resources: This is where you define the different components of your infrastructure, like servers, databases, and networks. You tell Terraform what you want each resource to look like and how it should be configured.
- Providers: Terraform works with different cloud providers like AWS, Google Cloud, Microsoft Azure or even Docker, and this section is where you specify which provider you’ll be using.
- Variables: Variables make your Terraform configuration more flexible. You can define variables that can be used throughout your configuration, making it easier to make changes in the future without having to modify the entire configuration file.
These are the basic building blocks of a Terraform configuration file, but as you get more familiar with Terraform, you’ll discover other features that can help you automate your infrastructure even more effectively.
Here’s an example of a basic main.tf Terraform configuration file using the local docker provider:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
# Set the required provider and version
terraform {
required_providers {
# Define the Docker Provider to be used and its version
# It is recommended to pin the version to ensure compatibility and avoid unexpected changes from new releases
docker = {
source = "kreuzwerker/docker"
version = "3.0.1"
}
}
}
# Configure the Docker Provider
provider "docker" {
}
# Create a Docker Image resource
# The `docker_image` resource will pull the latest version of the nginx image
# from the Docker hub, and keep a local copy of the image in the system.
resource "docker_image" "nginx" {
name = "nginx:latest"
keep_locally = true
}
# Create a Docker Container resource
# The `docker_container` resource will create and run a container from the latest version of the nginx image
# with the name "nginx". The container will expose port 8080 externally, and map it to port 80 internally.
resource "docker_container" "nginx" {
name = "nginx"
image = docker_image.nginx.image_id
ports {
external = 8080
internal = 80
}
}
In this example, the provider block specifies that we are using the Docker provider. The resource block pulls the “hello-world” Docker image. Finally, the output block displays a message to the console after the image has been pulled. With Terraform, you can automate the process of pulling Docker images and manage your Docker infrastructure as code.
After creating the Terraform configuration file, the next steps would be to initialize Terraform, validate the configuration, and then apply the changes.
Here are the basic steps to follow:
-
Initialize Terraform: Run the following command in the directory where the configuration file is located: terraform init. This will download the required providers and plugins.
1
terraform init
-
Validate the Configuration: Run the following command to validate the syntax and configuration file: terraform validate. This will check for any errors in the configuration file.
1
terraform validate
-
Plan the Changes: Run the following command to preview the changes that will be made to the infrastructure: terraform plan. This will give you a visual representation of the changes that Terraform will make to the infrastructure.
1
terraform plan --out=plan.out
-
Apply the Changes: Finally, run the following command to apply the changes to the infrastructure: terraform apply. This will create or modify the resources as defined in the configuration file.
1
terraform apply plan.out
It is important to carefully review the plan output before applying the changes, to make sure that the changes are exactly what you expect them to be.
4. Dive into Terraform State
4.1. Terraform State by definition
So what is Terraform State all about? Simply put, it’s a record of what Terraform knows about your infrastructure. It tracks changes made to your resources over time and helps Terraform understand how your real-world resources match up with what’s defined in your Terraform configuration.
Think of the Terraform State like a snapshot of your infrastructure. It has all the important details, like what resources you have and what their current values are. And it helps Terraform do its job by making sure that changes are made in the right order and resources aren’t accidentally destroyed or altered.
In short, Terraform State is a key component that makes Terraform work and helps you manage your infrastructure with confidence.
Every time Terraform is run, it records details about the infrastructure created in a file called the Terraform state file. By default, Terraform creates this file in the same folder as the Terraform execution, for example, if Terraform is run in the folder /app
, the state file will be located at /app/terraform.tfstate
. This file is formatted in a custom JSON format, which maps the Terraform resources defined in your configuration files to their corresponding representations in the real world. For instance, if your Terraform configuration includes:
After running terraform apply, here is a small snippet of the contents of the terraform.tfstate file (truncated for readability):
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
{
"version": 4,
"terraform_version": "1.3.7",
"serial": 32,
"lineage": "0bdc73b8-ebf4-abe3-9668-ecffa043b2fd",
"outputs": {},
"resources": [
{
"mode": "managed",
"type": "docker_image",
"name": "nginx",
"provider": "provider[\"registry.terraform.io/kreuzwerker/docker\"]",
"instances": [
{
"schema_version": 0,
"attributes": {
"build": [],
"force_remove": null,
"id": "sha256:3f8a00f137a0d2c8a2163a09901e28e2471999fde4efc2f9570b91f1c30acf94nginx:latest",
"image_id": "sha256:3f8a00f137a0d2c8a2163a09901e28e2471999fde4efc2f9570b91f1c30acf94",
"keep_locally": true,
"name": "nginx:latest",
"platform": null,
"pull_triggers": null,
"repo_digest": "nginx@sha256:6650513efd1d27c1f8a5351cbd33edf85cc7e0d9d0fcb4ffb23d8fa89b601ba8",
"triggers": null
},
"sensitive_attributes": [],
"private": "bnVsbA=="
}
]
}
],
"check_results": null
}
4.2. Managing the Terraform State
Managing Terraform state is an important aspect of using Terraform effectively. The state of your infrastructure is constantly changing, and Terraform needs to keep track of these changes to know what actions to take next. Here are a few key concepts and best practices related to Terraform state management:
- Storing State: You can store Terraform state in a local file or in a remote state backend like S3, Azure Blob Storage, or Terraform Cloud. It’s recommended to use a remote state backend in a production environment to keep your state data safe and easily accessible by multiple users.
- Locking State: To avoid conflicts, Terraform state can be locked so multiple users can’t make changes at the same time. This happens automatically when using a remote state backend, or can be done manually with tools like DynamoDB for local state.
- State Management Workflow: For best results, run terraform plan and terraform apply from the same state. If you make changes to your Terraform configuration, use terraform state push to update the remote state backend.
Backups: Regular backups are important, especially when using a remote state backend. You can make manual backups of the state file, or use a state management tool that includes backup functionality.
- To view the current state of the Terraform configuration:
1
terraform state show
- To move a resource to a different state in Terraform:
1
terraform state mv <current-address> <new-address>
- To delete a resource from the Terraform state:
1
terraform state rm <address>
- To import an existing resource into the Terraform state:
1
terraform import <address> <id>
- To refresh the state of a Terraform configuration:
1
terraform state refresh
- To output the Terraform state as a JSON file:
1
terraform state push -state-out=state.tfstate
- To import the Terraform state from a JSON file:
1
terraform state pull -state=state.tfstate
It’s important to note that Terraform state is a crucial aspect of managing infrastructure with Terraform, and it should be treated with care. Always make sure to back up the state file and keep it safe.
5. Conclusion
In conclusion, using Terraform with Docker can greatly simplify and automate the process of managing infrastructure and containers. By using Terraform’s HashiCorp Configuration Language to define the desired state of your infrastructure, you can deploy and manage containers, networks, and other resources with ease. Additionally, by utilizing Terraform’s state management features, you can ensure that your infrastructure remains in the desired state, even as changes are made over time. Whether you’re a beginner or an experienced user, the combination of Terraform and Docker provides a powerful solution for managing modern infrastructure and containers.