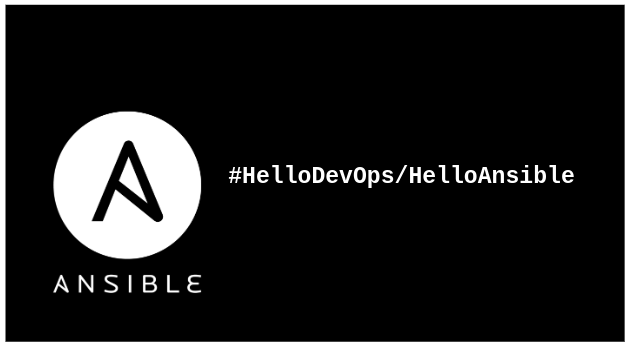
Welcome to the world of Ansible! If you’re a developer, devops engineer, IT architect or software engineer looking for an easy-to-use automation tool to help manage your IT infrastructure and applications, then look no further. Ansible is the perfect solution for automating routine tasks in your environment. In this blog post we will cover everything from installation of Ansible on different operating systems (Windows, Linux and macOS) as well as Docker containers, understanding its components and how it works along with some code examples that explain how best to use Ansible.
So grab a cup of coffee , fire up your favorite terminal
, and let’s get started with our HelloAnsible blog post!
1. Introduction
1.1 What is Ansible?
Ansible is an open-source automation tool that enables you to manage and configure your IT infrastructure through simple, declarative configuration files. It allows you to automate a wide variety of tasks, such as software deployments, package installations, configuration management, and file management, among others. Ansible is highly flexible, and it can be used with various IT infrastructure components, including servers, firewalls, switches, load balancers, and cloud services.
1.2 What are the benefits of using Ansible?
One of the unique features of Ansible is its agentless architecture, which means that it doesn’t require any additional software to be installed on the managed nodes. This results in a simplified setup process and makes it easier to scale and manage your infrastructure. Ansible also has a large and active community, which provides a wide range of pre-built modules and examples to help you get started quickly.
2. Installing Ansible
2.1. How to install Ansible on different operating systems
-
Linux: In most Linux distributions, Ansible can be installed using the package manager. Popular package managers such as apt, yum, and dnf can be used to install Ansible. For example, on Ubuntu, you can install Ansible by running the following command.
1 2
sudo apt-get update sudo apt-get install ansible
-
macOS: Installing Ansible on a macOS system is similar to installing on Linux. You can use the Homebrew package manager to install Ansible. To install Ansible on macOS, run the following command.
1 2
sudo apt-get update sudo apt-get install ansible
-
Windows: Installing Ansible on Windows requires additional setup. Ansible can be run on Windows using either Cygwin or the Windows Subsystem for Linux (WSL). The WSL is recommended for running Ansible on Windows as it provides a native Linux environment. To install WSL, follow these steps:
- Enable WSL on your Windows machine by following the Microsoft documentation.
- Install a Linux distribution through the Microsoft Store.
- After installing the Linux distribution, open the terminal window and run the following command to install Ansible:
1 2
sudo apt-get update sudo apt-get install ansible
2.1 How to install Ansible in Docker containers
To install Ansible in Docker containers, you can use a Dockerfile to build a container image that has Ansible installed. Here’s an example Dockerfile that installs Ansible on an Ubuntu container.
1
2
3
4
5
6
7
8
FROM ubuntu:latest
RUN apt-get update && \
apt-get install -y software-properties-common && \
apt-add-repository --yes --update ppa:ansible/ansible && \
apt-get install -y ansible
CMD ["ansible", "--version"]
You can build this Dockerfile using the docker build
command and then run the container to test Ansible.
Once the container is running, you can use the ansible
command to manage your infrastructure.
Note that running Ansible inside a Docker container can have limitations, especially when it comes to interacting with the host machine. Consider using volumes and network bridging to enable your Docker container’s privileged mode to run commands on the host machine.
3. Ansible Architecture
Ansible is a powerful automation tool that consists of several components, each with its own specific purpose. These components include playbooks, inventories, modules, roles, plugins and secrets.
3.1 Ansible Playbooks
Playbooks are the files that define the automation workflows in Ansible. Playbooks contain a set of one or more plays, and each play consists of a set of tasks that are executed sequentially. Playbooks can also include variables, conditionals, loops, and other control structures, allowing for complex and dynamic automation workflows.
Example of Ansible Playbooks: A playbook that installs and configures a web server on multiple hosts, a playbook that deploys an application to a Kubernetes cluster, a playbook that updates system packages and security settings across all servers in an infrastructure.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
- name: Install and configure web server on multiple hosts
hosts: webservers
tasks:
- name: Install httpd package
yum:
name: httpd
state: present
- name: Start and enable httpd service
service:
name: httpd
state: started
enabled: yes
- name: Copy web content
copy:
src: /path/to/web/content
dest: /var/www/html
- name: Set SELinux to permissive mode
seboolean:
name: httpd_can_network_connect
state: yes
- name: Deploy application to Kubernetes cluster
hosts: k8s_master
tasks:
- name: Deploy application
kubectl:
src: /path/to/app/deployment.yaml
state: present
- name: Update system packages and security settings across all servers
hosts: all
become: yes
tasks:
- name: Update system packages
yum:
name: '*'
state: latest
- name: Set timezone
timezone:
name: America/New_York
- name: Install and configure fail2ban
yum:
name: fail2ban
state: present
- name: Configure FirewallD rules
firewalld:
port: 80/tcp
permanent: yes
state: enabled
3.2 Ansible Inventories
Inventories are the files that define the infrastructure that Ansible manages. They contain a list of hosts and groups that Ansible uses to target specific systems for automation tasks.
Example of Ansible Inventories: An inventory that lists all the servers in a datacenter, an inventory that groups servers by location or role, an inventory that uses dynamic inventory sources like AWS or Azure.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
# Inventory that lists all servers in a datacenter
[datacenter]
server1.example.com
server2.example.com
server3.example.com
# ...
# Inventory that groups servers by location or role
[europe]
server1.example.com
server3.example.com
[us]
server2.example.com
server4.example.com
[web]
server1.example.com
server2.example.com
[db]
server3.example.com
server4.example.com
# Inventory that uses dynamic inventory sources
# AWS inventory
[aws:vars]
ansible_connection=aws
# Azure inventory
[azure:vars]
ansible_connection=azure
3.3 Ansible Modules
Modules are the building blocks of Ansible automation. They are reusable code blocks that perform specific tasks, such as managing packages, configuring services, or modifying files. Ansible comes with a large library of modules, and you can also create your own custom modules when needed.
Example of Ansible Modules: The apt module for managing package installations on Debian-based systems, the user module for managing user accounts and permissions, the systemd module for managing systemd-based services.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
- name: Install packages using apt module
apt:
name: ""
state: present
vars:
packages:
- apache2
- ssh
- name: Create user accounts using user module
user:
name: myuser
group: mygroup
state: present
- name: Manage systemd services using systemd module
systemd:
name: apache2
state: started
enabled: yes
3.4 Ansible Roles
Roles are a way to organize and reuse playbooks, variables, and other resources in Ansible. Roles are defined as a directory structure that contains playbooks, variables, and other files needed to perform a specific task or set of tasks.
Example of Ansible Roles: A role for managing a specific application or service, such as a MySQL or Apache role, a role for configuring network devices like routers or switches, a role for hardening system security settings.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
# Role for managing MySQL
- name: Install MySQL and create database
hosts: mysql
tasks:
- name: Install MySQL server
apt:
name: mysql-server
state: present
- name: Start MySQL service
service:
name: mysql
state: started
- name: Create MySQL database
mysql_db:
name: mydb
state: present
# Role for configuring network devices
- name: Configure Cisco routers
hosts: routers
tasks:
- name: Configure interface settings
ios_config:
lines:
- description This is a test interface
- ip address 10.0.0.1 255.255.255.0
parents: interface Ethernet0/0
register: output
- name: Save running configuration
ios_command:
commands: write mem
# Role for hardening system security settings
- name: Apply security hardening measures
hosts: all
become: yes
tasks:
- name: Set password policy
pam_limits:
limit_type: '@users'
hard: 'maxlogins 2'
- name: Disable root login via SSH
lineinfile:
path: /etc/ssh/sshd_config
regexp: '^PermitRootLogin'
line: 'PermitRootLogin no'
backup: yes
notify: restart sshd
- name: Update package repository cache
apt:
update_cache: yes
3.5 Ansible Plugins
Plugins are extensions to Ansible that add additional functionality or integrations with other tools and systems. Ansible supports various plugins for things like authentication, inventory sources, and notification services.
Example of Ansible Plugins: A plugin for integrating with a source control management system like Git or SVN, a plugin for sending notifications to messaging services like Slack or Microsoft Teams, a plugin for managing cloud infrastructure like AWS or GCP.
1
2
3
4
5
6
7
8
- name: Install SCM source control plugin
ansible-galaxy collection install community.general
- name: Install Slack notification plugin
ansible-galaxy collection install community.general
- name: Install AWS plugin
ansible-galaxy collection install amazon.aws
3.6 Ansible Secrets
Ansible Secrets is a feature built into Ansible for encrypting sensitive data such as passwords, certificates, and keys. Ansible Secrets, also referred to as Ansible Vault, provides a secure and easy way to manage secrets within Ansible projects, and it can be used to encrypt individual or multiple files containing sensitive data. The encrypted data is decrypted automatically when the playbook is run within the environment, ensuring that sensitive information is always kept secure. The use of Ansible Secrets is an important part of any playbook that handles sensitive information, and its implementation is a key step towards ensuring secure infrastructure for your projects.
Example of Ansible Secrets: For example, if you have an Ansible playbook that provisions a server and sets up a database, you might need to include credentials for the database as part of the playbook. You could store these credentials in an encrypted Ansible Vault file, along with any other sensitive data that is required for the task.
Here’s an example of how you might use Ansible Secrets to manage database credentials
- Create an encrypted file for your credentials using the ansible-vault create command
1
ansible-vault create credentials.yml
- Add your sensitive data to the file and save it
1
2
db_username: myuser
db_password: MyP@ssw0rd
- In your Ansible playbook, use the include_vars command to load the credentials file
1
2
- name: Include credentials
include_vars: credentials.yml
- Use the loaded variables in your playbook tasks
1
2
3
4
5
6
7
- name: Create database user
mysql_user:
name: ""
password: ""
priv: "*.*:ALL"
host: "localhost"
state: present
With this setup, your sensitive data remains protected even if your playbook is shared or stored in source control.
4. Best Practices
When writing Ansible code, it’s important to follow best practices to ensure that your code is clean, maintainable, and efficient. Here are some best practices for using Ansible:
- Use consistent naming conventions: Consistent naming for host groups, variables, plays, and tasks makes your code easier to read and maintain.
- Use native YAML syntax: Avoid shortcuts that can make code harder to read and debug.
- Use modules: Modules have built-in error handling and are idempotent, making them a better choice than shell or command scripts.
- Use roles: Group related tasks into roles and execute them across different playbooks. You can also use Ansible Galaxy to find and share roles.
- Use tags: Use tags to organize and manage plays and tasks, grouping similar tasks together.
- Use variables and templates: Streamline your code using variables and templates, making it more reusable and easier to modify.
- Use Ansible Collections: Use Ansible Collections to save time and improve the consistency of your code.
By following these best practices, you can write clean, organized, and efficient Ansible code that is easy to read, modify, and maintain. Additionally, by using Ansible’s built-in features and capabilities, such as roles, modules, and collections, you can save time and improve the overall quality of your automation processes.
In the other hand, there are also common mistakes that users can easily make when using Ansible, which can lead to issues with their automation processes. Here are some of the most common mistakes to avoid when using Ansible:
- Using loops with conditional statements: When using loops with conditional statements, you need to pay close attention to the order of the statements in your playbook. This can lead to unexpected behavior if not done correctly.
- Not specifying hosts or groups: When running playbooks, make sure to specify the hosts or groups that the playbook targets. Failing to do so can cause unintended actions on the wrong hosts or groups.
- Not testing your code: It’s crucial to test your Ansible playbooks thoroughly to ensure they work as expected. Testing helps you catch errors and ensure that your automation processes run smoothly.
By avoiding these common mistakes and following best practices when working with Ansible, you can write clean, organized, and efficient automation code that saves time and makes managing your infrastructure more manageable.
5. Conclusion
In conclusion, Ansible is a powerful open-source automation tool that offers numerous benefits for IT professionals. It allows you to automate IT tasks, increasing efficiency and productivity, and reducing the risk of errors caused by manual processes.